- ์ด๋ฒ ๊ธ์์๋ Socket.IO๋ฅผ ์ฌ์ฉํด ๊ฒ์๋ฌผ๊ณผ ๋๊ธ์ ์ข์์๋ฅผ ์ถ๊ฐํ๋ฉด ์ค์๊ฐ์ผ๋ก ์๋ฆผ์ ๋ณด๋ด๋ ๊ธฐ๋ฅ์ ๊ตฌํํจ.
- ํด๋ผ์ด์ธํธ๋ ์ฆ์ ์ข์์๊ฐ ๋ฐ์๋ ์ ๋ณด๋ฅผ ๋ฐ์ ์ ์์.
1. ์๋ฒ ์ฝ๋
1) ๊ธฐ์กด์ server.js ์์
- ์๋ฒ์์ Socket.IO๋ฅผ ์ด๊ธฐํํ๊ณ ํด๋ผ์ด์ธํธ์์ ์ฐ๊ฒฐ์ ๊ด๋ฆฌ.
- ์ข์์๊ฐ ๋ฐ์ํ๋ฉด like-post์ like-comment ์ด๋ฒคํธ๋ฅผ ํด๋ผ์ด์ธํธ์๊ฒ ์ ์กํฉ๋๋ค.
// server.js
const socketIo = require("socket.io");
let io;
function initSocket(server) {
io = socketIo(server);
io.on("connection", (socket) => {
console.log("A user connected");
socket.on("disconnect", () => {
console.log("User disconnected");
});
});
}
function emitComment(data) {
if (!io) {
console.error("Socket.io is not initialized.");
return;
}
io.emit("new-comment", data); // ์ด๋ฒคํธ ๋ฐ์
console.log("Emitted new-comment event:", data); // ์ด๋ฒคํธ ๋ฐ์ ํ ๋ก๊ทธ ์ถ๊ฐ
}
function emitCommentLike(data) {
if (!io) {
console.error("Socket.io is not initialized.");
return;
} else {
io.emit("like-comment", data);
console.log("Emitting like-comment event:", data);
}
}
function emitPostLike(data) {
if (!io) {
console.error("Socket.io is not initialized.");
return;
} else {
io.emit("like-post", data);
console.log("Emitting like-post event:", data);
}
}
module.exports = { initSocket, emitComment, emitCommentLike, emitPostLike };
2) ๊ธฐ์กด์ likePost.js์ likeComment.js ์์ (์ด๋ฒคํธ ๋ฐ์)
- ๊ฒ์๋ฌผ๊ณผ ๋๊ธ์ ์ข์์๊ฐ ๋๋ฆฌ๋ฉด, ๊ฐ๊ฐ emitLikePost์ emitLikeComment ํจ์๊ฐ ํธ์ถ๋์ด Socket.IO๋ฅผ ํตํด ๊ด๋ จ ์ ๋ณด๋ฅผ ํด๋ผ์ด์ธํธ๋ก ์ ์ก
likePost.js
// likePost.js
const { emitLikePost } = require("../../server");
router.post("/:postId/like", auth, async (req, res) => {
const postId = req.params.postId;
const userId = req.user._id;
try {
const post = await Post.findById(postId);
if (!post) {
return res.status(404).json({ message: "๊ฒ์๋ฌผ์ ์ฐพ์ ์ ์์ต๋๋ค." });
}
// ์ด๋ฏธ ์ข์์๊ฐ ๋๋ ค์๋ค๋ฉด ์ข์์ ์ทจ์
const index = post.likes.indexOf(userId);
if (index > -1) {
post.likes.splice(index, 1); // ์ข์์ ์ทจ์
} else {
post.likes.push(userId); // ์ข์์ ์ถ๊ฐ
}
await post.save();
emitLikePost({
postId: postId,
userId: userId,
likeCount: post.likes.length,
});
return res.status(200).json({
message: "์ข์์๊ฐ ์ฑ๊ณต์ ์ผ๋ก ์ฒ๋ฆฌ๋์์ต๋๋ค.",
likeCount: post.likes.length,
});
} catch (error) {
return res.status(500).json({
message: "์ข์์ ์ฒ๋ฆฌ ์ค ์ค๋ฅ๊ฐ ๋ฐ์ํ์ต๋๋ค.",
error: error.message,
});
}
});
module.exports = router;
likeComment.js
// likeComment.js
const { emitLikeComment } = require("../../server");
router.post("/:commentId/like", auth, async (req, res) => {
const commentId = req.params.commentId;
const userId = req.user._id;
try {
const comment = await Comment.findById(commentId);
if (!comment) {
return res.status(404).json({ message: "๋๊ธ์ ์ฐพ์ ์ ์์ต๋๋ค." });
}
// ์ด๋ฏธ ์ข์์๊ฐ ๋๋ ค์๋ค๋ฉด ์ข์์ ์ทจ์
const index = comment.likes.indexOf(userId);
if (index > -1) {
comment.likes.splice(index, 1); // ์ข์์ ์ทจ์
} else {
comment.likes.push(userId); // ์ข์์ ์ถ๊ฐ
}
await comment.save();
emitLikeComment({
commentId: commentId,
userId: userId,
likeCount: comment.likes.length,
});
return res.status(200).json({
message: "๋๊ธ ์ข์์๊ฐ ์ฑ๊ณต์ ์ผ๋ก ์ฒ๋ฆฌ๋์์ต๋๋ค.",
likeCount: comment.likes.length,
});
} catch (error) {
return res.status(500).json({
message: "๋๊ธ ์ข์์ ์ฒ๋ฆฌ ์ค ์ค๋ฅ๊ฐ ๋ฐ์ํ์ต๋๋ค.",
error: error.message,
});
}
});
module.exports = router;
2. ํด๋ผ์ด์ธํธ ์ฝ๋ (์์)
// client.js
const io = require("socket.io-client");
// ์๋ฒ์์ ์์ผ ์ฐ๊ฒฐ
const socket = io("http://localhost:3000"); // ์๋ฒ URL์ ๋ง๊ฒ ์์
// ์๋ฒ์ ์ฐ๊ฒฐ ์ฑ๊ณต ์
socket.on("connect", () => {
console.log("์๋ฒ์ ์ฐ๊ฒฐ๋์์ต๋๋ค.");
});
// ์๋ฒ์์ "new-comment" ์ด๋ฒคํธ ์์
socket.on("new-comment", (data) => {
console.log("์๋ก์ด ๋๊ธ์ด ๋ฌ๋ ธ์ต๋๋ค!");
console.log(data); // ์๋ฒ๋ก๋ถํฐ ๋ฐ์ ๋ฐ์ดํฐ ์ถ๋ ฅ
});
// ์๋ฒ์์ "like-comment" ์ด๋ฒคํธ ์์
socket.on("like-comment", (data) => {
console.log("๋๊ธ์ ์ข์ํฉ๋๋ค!");
console.log(data); // ์๋ฒ๋ก๋ถํฐ ๋ฐ์ ์ข์์ ์๋ฆผ ๋ฐ์ดํฐ ์ถ๋ ฅ
});
// ์๋ฒ์์ "like-post" ์ด๋ฒคํธ ์์
socket.on("like-post", (data) => {
console.log("๊ฒ์๋ฌผ์ ์ข์ํฉ๋๋ค!");
console.log(data); // ์๋ฒ๋ก๋ถํฐ ๋ฐ์ ์ข์์ ์๋ฆผ ๋ฐ์ดํฐ ์ถ๋ ฅ
});
3. ๊ฒฐ๊ณผ ๐
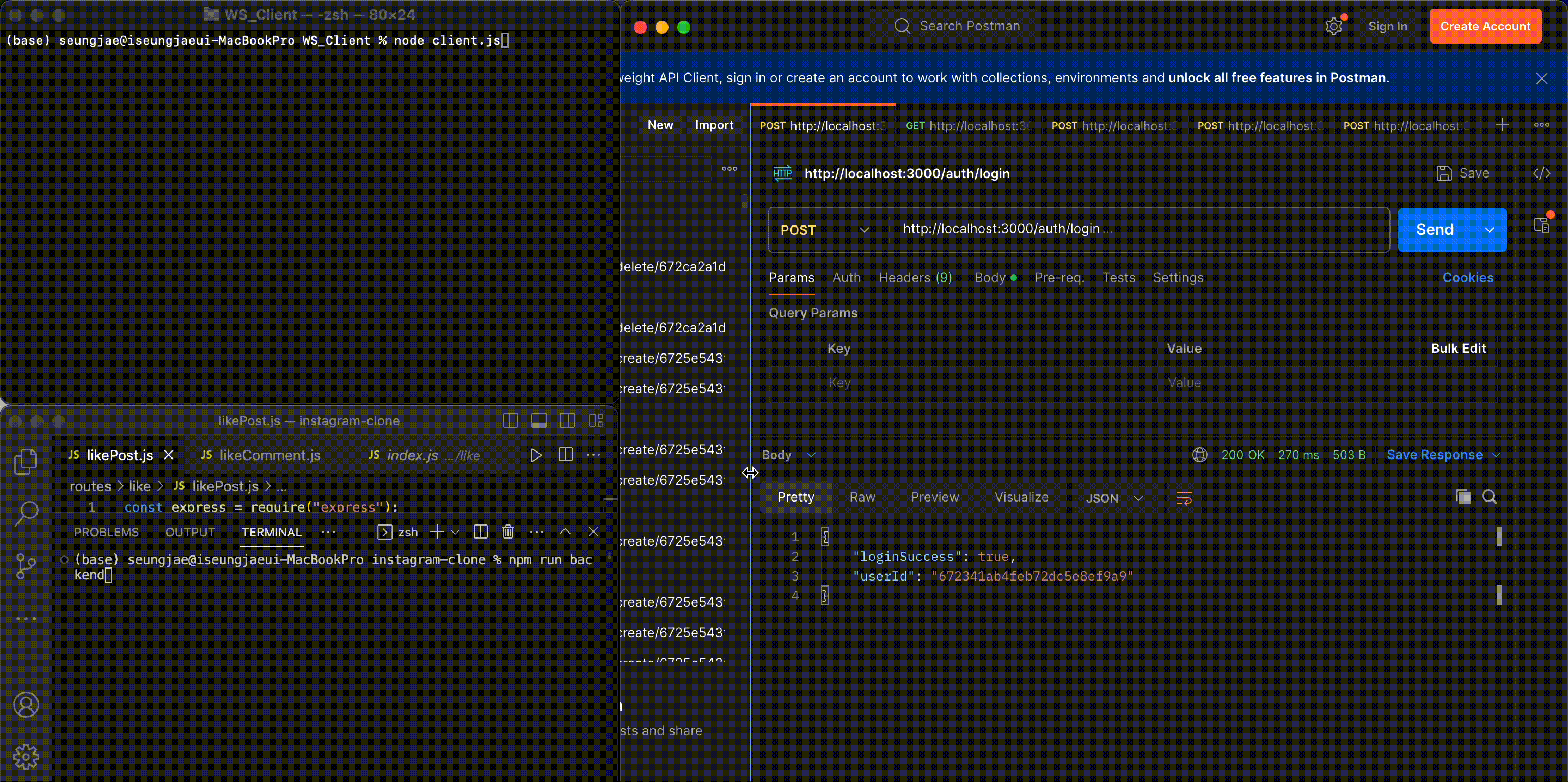